题意:
每个物体都有一个权值a[i],求出的是背包目前sum值不能再放入任何一个物体,意思就是sum+a[i] <= d这个的方案数
想法:
先排序,sum[i],前i个物体的权值和
可以枚举1-N那个物体不在背包的,这样子i之前的全都在背包里,在i+1-N的进行背包,背包的大小在d-sum[i]到d-sum[i-1]+1之间,相想为何要+1
然后从N开始向下枚举,改一改如上算法就行
1 | /* From: Lich_Amnesia |
题意:
每个物体都有一个权值a[i],求出的是背包目前sum值不能再放入任何一个物体,意思就是sum+a[i] <= d这个的方案数
想法:
先排序,sum[i],前i个物体的权值和
可以枚举1-N那个物体不在背包的,这样子i之前的全都在背包里,在i+1-N的进行背包,背包的大小在d-sum[i]到d-sum[i-1]+1之间,相想为何要+1
然后从N开始向下枚举,改一改如上算法就行
1 | /* From: Lich_Amnesia |
简单递推,记得记录路径
dp[i][j] = max(dp[i-1][j-1] + a[i][j], dp[i][j-1])
可能会出现
3 5
-50 -50 -50 -50 -50
-50 -50 -50 -50 -50
-50 -50 -50 -50 -50
这样的数据
记得初始化好
1 | /* From: Lich_Amnesia |
注意判定是逆时针旋转扫描边就行(这个还不是很清楚,check函数)
每次走的时候选择最左的
1 | /* From: Lich_Amnesia |
题意:有n个人排成一列,给出前k个人的数字,从第k+1个人开始,给他们分配数字,分配的方法是取当前没有出现过的最小正整数,例如8人,前3人的数字为3 5 8,第4到第8个人的数字分别为1 2 4 6 7,整个序列为3 5 8 1 2 4 6 7
数据很大,不可能模拟
1.留意到一点,因为是取最小的没用过的正整数,所以我们可以先把所有可能用的正整数保存下来,按区间保存
2.例如已经用了3 5 8,没用的就是
[1,2] 2个
[4,4] 1个
[6,8] 3个
现在
要为第k+1个人分配数字,那么可以立刻知道是分配1,因为1是第1个没有用过的
要为第k+5个人分配数字,可以立刻知道是7,因为7是第5个没有用过的数字
所以要为第m个数字分配数字,在上面没有用过的数字表里面找,先找到它在哪一块,再找到是哪一块的第几个,其中找在哪一块,用二分即可
1 | /* From: Lich_Amnesia |
二分答案
假设当前二分的答案为 t,那么:
对于ai <= t的衣服,显然让它们自然风干就可以了。
对于ai > t的衣服,我们需要知道该衣服最少用多少次烘干机。
设该衣服用了x1分钟风干,用了x2分钟烘干机。
那么有 x1 + x2 = t 和 ai <= x1 + x2 * k,联立两式可得 x2 >= (ai - t) / (k - 1),即最少使用次数为[(ai - t) / (k - 1)] 的最小上界。
最后,判断一下总使用次数是否少于 t 即可。
记得不要除零
1 | /* From: Lich_Amnesia |
同2167,只不过这次不是单位圆覆盖了,是给定半径了
1 | /* From: Lich_Amnesia |
先上一个O(n^3)的算法
也就是枚举两个距离小于2的点,用它们来固定一个圆,当然对称圆也要算,再枚举所有点,看是不是在这个圆内,当然这题这样就可以水过,不过这并不是最优的。
注意ans至少为1个.
1 |
|
还有一个O(n^2 log n)的算法,这种方法其实也简单,先枚举一个点,再枚举所有与它距离小于2的点(保证两圆相交),这样就可以求出相交弧,对于每一个圆把所有弧保存下来(只保存弧上的那两个端点一个设为-1一个设为1),并离散化(把端点存到数组里面,然后按照极角排序),这样从极角最小的开始扫,直到扫到端点对数达到这个圆上的弧的个数为止,每次扫描从1开始,标记这个弧开始了sum++,然后直到扫到这个弧结束扫到-1,这时sum—,相当于区间最大和。
就能算出覆盖次数最多的一段弧,这个次数也就是答案了,具体看代码吧
1 |
|
这个问题最初表述为:
你有一个天平秤和12个硬币,其中1个是假的。假冒重量比其他硬币少或者多。你能确定假币是哪一枚,并判断它是重还是轻?
较难并且较为普遍的问题是:
对于一些给定的n>1,有(3^ N - 3)/2个硬币,其中1是假冒的。假冒重量比其他硬币少或者多。你能用天平秤,确定伪造硬币,并判断它是重还是轻?
对于 n = 2, 总共3个硬币,称重步骤如下:
1 against 2
1 against 3
对于每一次称重都有三种结果:倾向left(l),倾向right(r),或者balance(b)。如下表格给出了对于每种结果的答案:
l l 1 too heavy
l b 2 too light
l r (not possible)
b l 3 too light
b b no fake coin
b r 3 too heavy
r l (not possible)
r b 2 too heavy
r r 1 too light
其中 l r 以及 r l 是永远不会出现的结果。
现在,对于n = 3,我们替换原先一个硬币c换成3c-2,3c-1以及3c。按照上面所说的方法:
1 2 3 against 4 5 6
1 2 3 against 7 8 9
这样两次之后,我们就知道哪一个集合({1,2,3}, {4,5,6} ,{7,8,9})里面有那个假冒的硬币,并且可以知道假冒的硬币是重了还是轻了。再通过一次称重操作,我们就可以确定哪一个是假冒的硬币,只要每一个集合取一个放在左边,每一个集合取一个放在右边:
1 4 7 against 2 5 8
但是这样只能解出1到9的结果,总共有12 (= (3^3 - 3)/2)个。考虑到结果集合里不会出现 “l r” 以及”r l”(上面的两个123的称重方法)。可以利用这个条件,把10,11, 12,放进式子里:
1 2 3 10 against 4 5 6 11
1 2 3 11 against 7 8 9 10
1 4 7 10 against 2 5 8 12
这样如果不是如下情况的话,假冒的就是在1-9,否则就是在10-12里面的。根据结果分析得出:
l r l 10 too heavy
l r b 11 too light
l r r (not possible)
b b l 12 too light
b b b no fake coin
b b r 12 too heavy
r l l (not possible)
r l b 11 too heavy
r l r 10 too light
另外的一种解决的方案:
1 4 6 10 against 5 7 9 12 2 5 4 11 against 6 8 7 10 3 6 5 12 against 4 9 8 11
对于这个方案,”l l l”与”r r r”并不可能。
可以考虑这个问题,有多少不同方案?可以确定假冒的硬币,并且确定是重了还是轻了
现在我们可以考虑一下如何解决n = 4的情况。同理,我们替换原先一个硬币c换成3c-2,3c-1以及3c。这样可以解决36的硬币,现在还差3个。现在我们还是按照上面的两种结果是不可能的”l r r”与”r l l”,于是我们又按照上次的方法来加入三个硬币:
........... 37 against ........... 38 ........... 38 against ........... 37 ........... 38 against ........... 37 1 4 7 .. 34 38 against 2 5 8 .. 35 39
如此推断,对于任意的n > 1,我们都能用n次称重找到那个假冒的硬币。
在TED上看到他StephenWolfram的一个演讲,有关A new kind of science,通过对所有知识的计算来分析出一定的结论。根据已知知识来推算未知部分,是人工智能的一部分,但是一般所能想到的比如根据已知蛋白质序列推出未知序列的结构。
但是他所想的却不一样,在计算空间之下,利用简单的规则组合成复杂形式,探讨我们这个物理世界的本质。或者是通过简单音符的组合来进行歌曲的创作。
现在已经做出来的[http://www.wolframalpha.com/](http://www.wolframalpha.com/)已经可以做到进行实时的知识检索以及分析了,实在是太nice了。把所有已知知识进行汇总计算,推导出来的是不是就是原来未知的东西,那岂不是就可以探寻本质了么?非常佩服~
有关wolfram alpha 请戳:[http://zh.wikipedia.org/wiki/Wolfram_Alpha](http://zh.wikipedia.org/wiki/Wolfram_Alpha)
还有在这之前建立的数学检索库: [http://mathworld.wolfram.com/](http://mathworld.wolfram.com/)
以下附上那篇TED演讲以及有关论文
链接:[http://pan.baidu.com/s/1dDtGGM1](http://pan.baidu.com/s/1dDtGGM1) 密码:ip16
在使用Word办公时,我们经常需要批量查找替换,但是直接进行文字替换时,经常是达不到我们期望的效果,这时我们就需要增加一些通配符来查找了。下面给大家列出Word查找/替换栏代码·通配符一览表,具体的实例我们将在以后的文章中陆续介绍。例如,Word查找替换总是反引号,怎么办?
Word 查找栏代码·通配符一览表
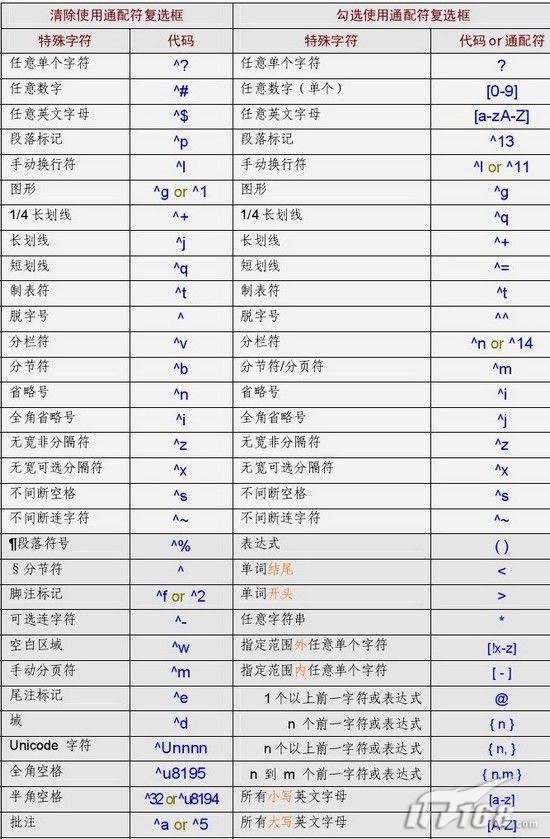 |
图1 通配符 |
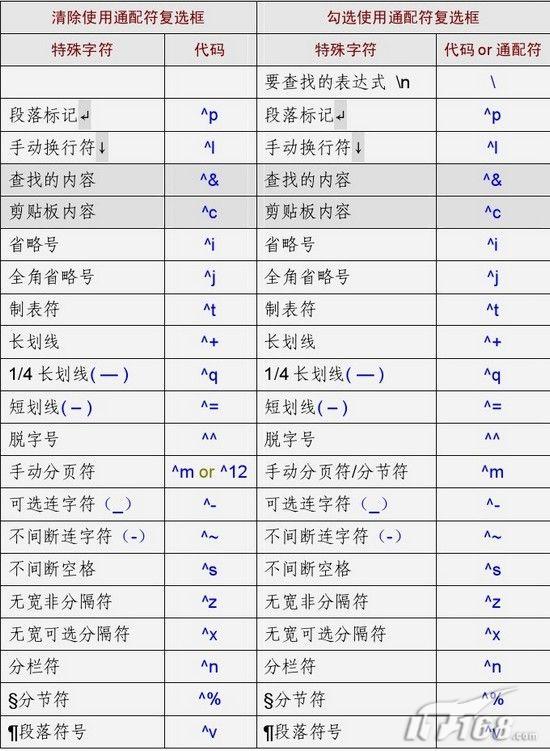 |
图2 通配符 |
注:要查找已被定义为通配符的字符,该字符前键入反斜杠 。查找?、*、(、)、[ 、] 等的代码分别是?、*、(、)、[、] 。